New Neuron Popup System
We have replaced window.alert
, window.confirm
, and window.prompt
with a custom popup system. This provides better control and accessibility for user interactions.
Overview
- Improved User Experience: The popups have a consistent style and can be customized.
- Accessibility: Focus trapping ensures proper keyboard navigation.
- Better Control: Supports handling of modal dialogs with promises.
How to use it
The popup system is located in the Master.js
file. To use it include the folowing headers in your markdown or master file.
Javascript: /AlertPopup.md.js
Javascript: /ConfirmPopup.md.js
Javascript: /PromptPopup.md.js
Javascript: /Master.js
You also need a dialog container in the bottom of your html body.
<div id="native-popup-container"></div>
Additionally, the theme used must implement the base theme base.cssx
for styling. Alternatively you could style it yourself, but it would be recomended to instead cascade over the already existing css or changing the themes cssx variables;
Usage
Alert
Use Alert(message)
to display a basic alert popup.
const popup = PopupHandler();
await popup.Alert("This is an alert message.");
Confirm
Use Confirm(message)
to display a confirmation dialog that returns a boolean.
const result = await popup.Confirm("Are you sure?");
if (result) {
console.log("User confirmed.");
} else {
console.log("User canceled.");
}
Prompt
Use Prompt(message)
to capture user input.
const userInput = await popup.Prompt("Enter your name:");
console.log("User entered:", userInput);
Details
- All popups are contained in a shared container.
- The popup backdrop is generated by the Master.js file, and placed at the bottom of the body tag
- If you initiate a new popup whilst one is already open, it will cover the old one. The old one will be accesible after you interact with the newly created one. You can think of the popups in a stack datastructure.
- Focus trapping disables focusing outside the popup using tab and shift + tab.
- Your master markdown file must include the following element at the bottom to contain the popups:
- If you dont need to wait for an Ok press when using Alert() you don’t need to await the response.
Benchmark: Javascript framework for communicating with server
When Communicating with a server from Javascript code, you have different options for communicating with the server: You have the oldest technology: XML HTTP Request Object (or XHR), you have the more modern Web API Fetch, or you can use a proprietary API, such as the Google Axios library. They all have their pros and cons. But which of these is most efficient from a performance perspective? From a server’s point of view, it matters, little, as communication is performed using HTTP or Web Sockets in all cases. From a client’s point of view, it depends on the browser. Older browser do not support modern technologies. More modern technologies are easier to use during development. Performance also depends on how well the different technologies are supported and optimized on that platform (and the computer you run the tests on and the network it is connected to).
The site measurethat.net has a benchmark test that measures these technologies. You can test it by clicking on the link. The benchmark returns the following results on different browsers:
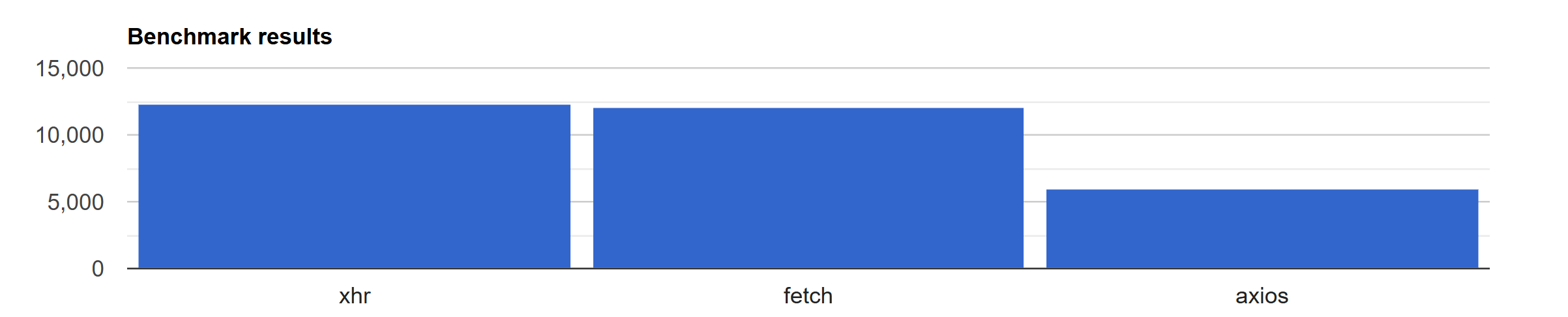
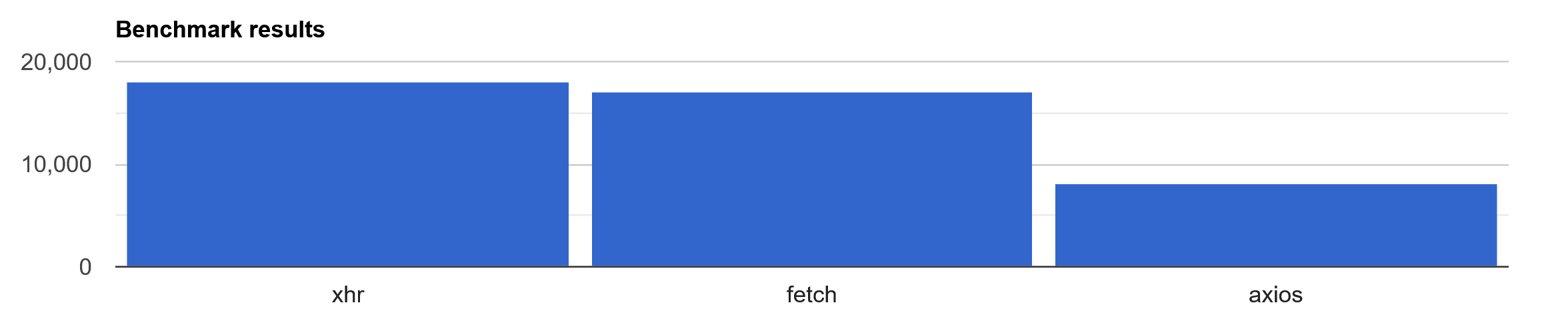

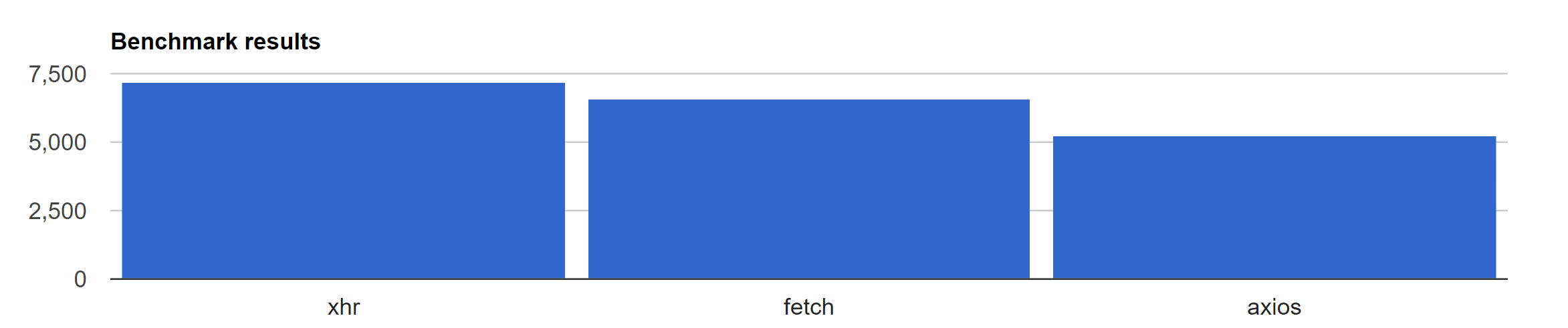
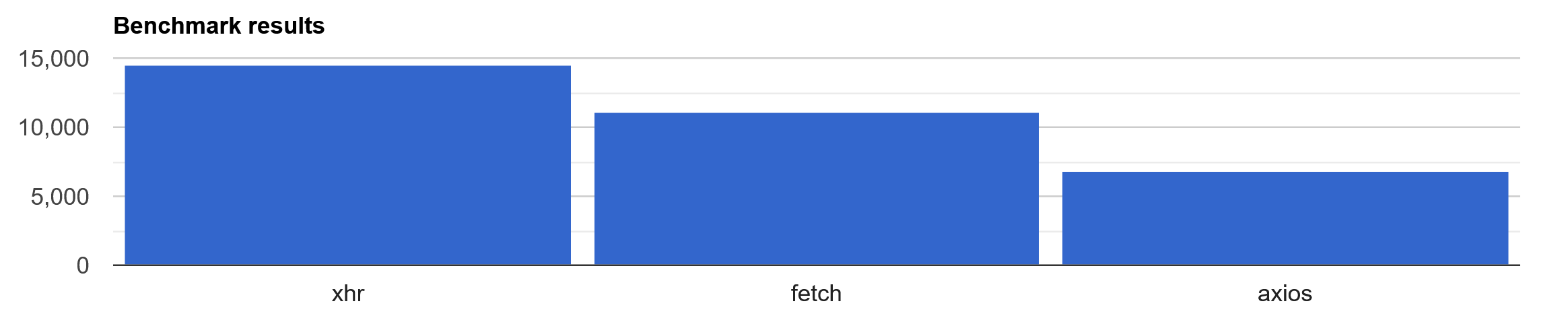
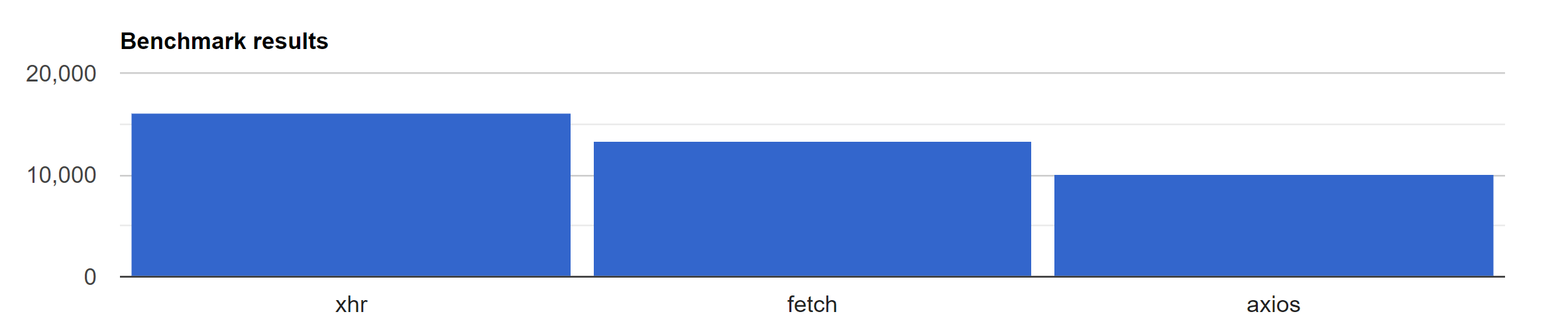
Any browsers of interest missing from the list? Let me know.
Generation of JavaScript
The Markdown engine can generate JavaScript for you automatically, facilitating the dynamic creation of items on web pages. This conversion can be done either by specifying an Accept: application/javascript
header when requesting for a Markdown file, or by referring to a Markdown file with an additional .js
extension after the traditional .md
extension. Referring this way to a file Test.md.js
for instance, will generate a JavaScript rendering of the Test.md
file. This method is useful if referring to JavaScript files from the header of an HTML file, for instance, where you cannot control the browser’s selection of HTTP Accept header field in the request.
When converting a Markdown file (for example Test.md
) to JavaScript (for example Test.md.js
), two functions are created and included in the file:
function CreateHTMLTest(Args);
function CreateInnerHTMLTest(ElementId, Args);
The final Test
in the funcion names are taken from the name of the Markdown file being converted. This makes it possible to include multiple JavaScript files generated from multiple Markdown files on the same page. The Args
argument can be used to send information to the function, which is later used by inline script when generating HTML.
The first function returns a string containing the HTML generated by the JavaScript. The second function calls the first function to generate HTML, the looks in the DOM of the page to find an element with a given id
attribute, and then sets the innerHTML
property of that element to the generated HTML.
Things to keep in mind when converting Markdown to JavaScript:
Script placed between double braces
{{
and}}
is preprocessed on the server and affect the structure of the Markdown, which in turn affects the generated JavaScript. Such script do not have access to theArgs
argument. Instead they have access to any session variables that may exist.Script placed between single braces
\{
and\}
is not processed on the server at all. Instead, it is assumed to be JavaScript itself, and inserted as-is into the JavaScript. This allows you to populate the dynamic HTML using values from your browser, without having to request the server to do it. This also means, that the script syntax normally used for single-braces evaluation on the server, is not used in the JavaScript case. The inline script has access toArgs
, but as it runs in the browser, does not have access to server-side session-state variables.If the Markdown only contains a header-less table (i.e. a table with zero header rows), the JavaScript rendered will only generate the table rows, not the surrounding
table
,thead
andtbody
elements, to facilitate dynamic addition of rows to a table.
Generated JavaScript Example
Consider the following Markdown page, saved as Test.md
:
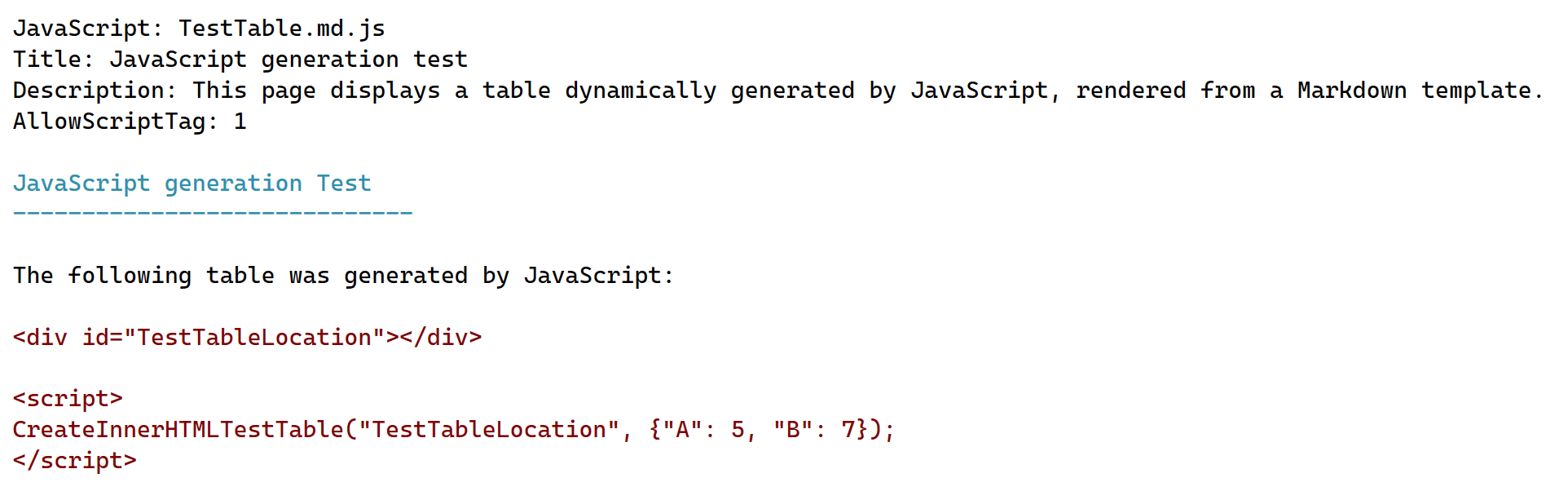
It refers to a Markdown template called TestTable.md
, in a JavaScript header. To make sure the server converts this Markdown to JavaScript, the extension .js
is added to the filename, resulting in a reference to TestTable.md.js
file. When the browser requests this file, the server recognizes that the file does not exist. Instead, it recognizes the .js
extension, understands that it refers to the Accept: application/javascript
header, and modifies the request to refer to TestTable.md
with the corresponding Accept
header set. The server then loads the Markdown, converts it to JavaScript, and returns JavaScript that generates HTML from the following Markdown template:
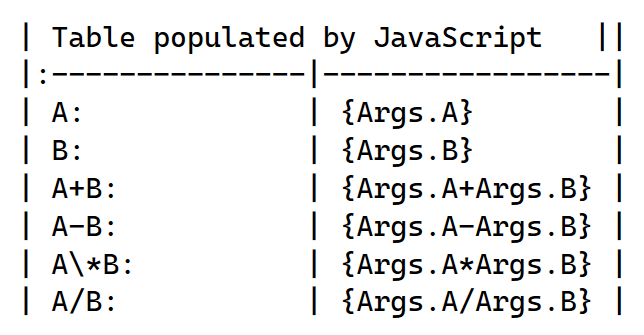
This will generate a page similar to:
JavaScript generation Test
The following table was generated by JavaScript:
Table populated by JavaScript | |
---|---|
A: | 5 |
B: | 7 |
A+B: | 12 |
A-B: | -2 |
A*B: | 35 |
A/B: | 0.7142857142857143 |
Agent API Javascript NPM package
Currently, there is no package on the public npm package registry, but you can still add the package as a dependency in your package.json. You just add
“agent-api”: “https
/github.com/Trust-Anchor-Group/AgentApiJavascript.gitnpm-package”
to your dependencies. Though, note that this might require that you have the newest version of the AgentApi on your neuron (which may require the newest neuron version). This is because the repo is designed for the latest version, which means that it might be different since the AgentApi is ever evolving. And at last, there currently is only one version, which if it is updated, and you later install your dependencies, the package might have changed. So if you for some reason do not plan to update the neuron or your code, it might be better to copy the code in the package. For those curious, there is currently not a package for the typescript version.
In The Code
if you use ES6 import syntax just use : `js import AgentAPI from "agent-api" AgentApi.Legal.CreateContract()
or if you use CommonJs: `js const AgentAPI = require("agent-api") AgentApi.Legal.CreateContract()
The Github Repository
As you may see, the package is located in the npm-package branch on the AgentApiJavascript repository on Github. If you notice that the main branch have got some important commits that the npm-package does not, please consider rebasing the npm-package branch to the main branch. If you are not sure how to rebase, consider learning more about it, absolutely use something like Sourcetree to check that you did it right, and if you are worried, make a backup of the repo.
Posts tagged #javascript
No more posts with the given tag could be found. You can go back to the main view by selecting Home in the menu above.