Notarius Electronicus
A new white-paper is available: Notarius Electronicus, outlining the importance of the Trust Provider in an Open and Secure Smart Society
Abstract. There are different strategies to create decentralized open networks that are secure at the same time. Some are based on distrust and often rely on costly algorithms such as Proof of Work or Proof of Stake to prove actor’s in-tentions are not malicious, followed by a consensus method that determines the true state of the network. Other methods rely on trust, trust relationships, digital identities or identifiers and digital signatures to prove claims made. This paper describes the use of a “Notarius Electronicus” as a digital representation of a “Notarius Publicus” used in the real world, as a means to create Trust in an open digital network, digitally mirroring how we have created Trust in our open analog societies for millennia.
Processing signed contracts in a state machine: Open Vote example
The LegalLab repository contains a new example that illustrates how Neuro-Feature token state machines can be used to process signed smart contracts. The example implements a simple open voting system that tokenizes each vote, counting ballots within a given time frame, as they are cast. Each ballot is a smart contract signed by a participant. The state-machine of the vote counts accepted ballots, rejects incorrect ballots, and logs events to the Neuro-Ledger for transparency and auditability.
Note: The same architecture as exemplified by the Open Vote set of contracts (Vote + Ballot contracts) can be used in many technically similar (albeit conceptually different) cases. One such example is the tokenization of agriculture, for example, where each cultivation can be tokenized, and it can keep track of its current state transparently (for the end consumer) by processing observations, each observation recorded as a signed smart contract with information about what has occurred. Another example can be the tokenization of medical journals, for interoperability and privacy protection. The journal observes diagnoses and tests being performed, each one recorded as a signed smart contract with the appropriate information.
Vote Contract
The first contract in the Open Voting model (OpenVoteYesNoAbstain.xml
), contains the state-machine Neuro-Feature token definition and contract. It defines the basic states of ballot processing. It assumes each ballot contains machine-readable information, as defined by the schema OpenVote.xsd
, also downloadable online via its target namespace: https://paiwise.tagroot.io/Schema/OpenVote.xsd
.
Note: Each contract containing machine-readable instructions will only be accepted if each Neuron® can validate the XML it contains. This is done by downloading the schema files from the corresponding target namespaces and using these schemas for validation. If the validation does not complete successfully, the contract is automatically rejected.
The states defined in the contract can be illustrated with the following state diagram:
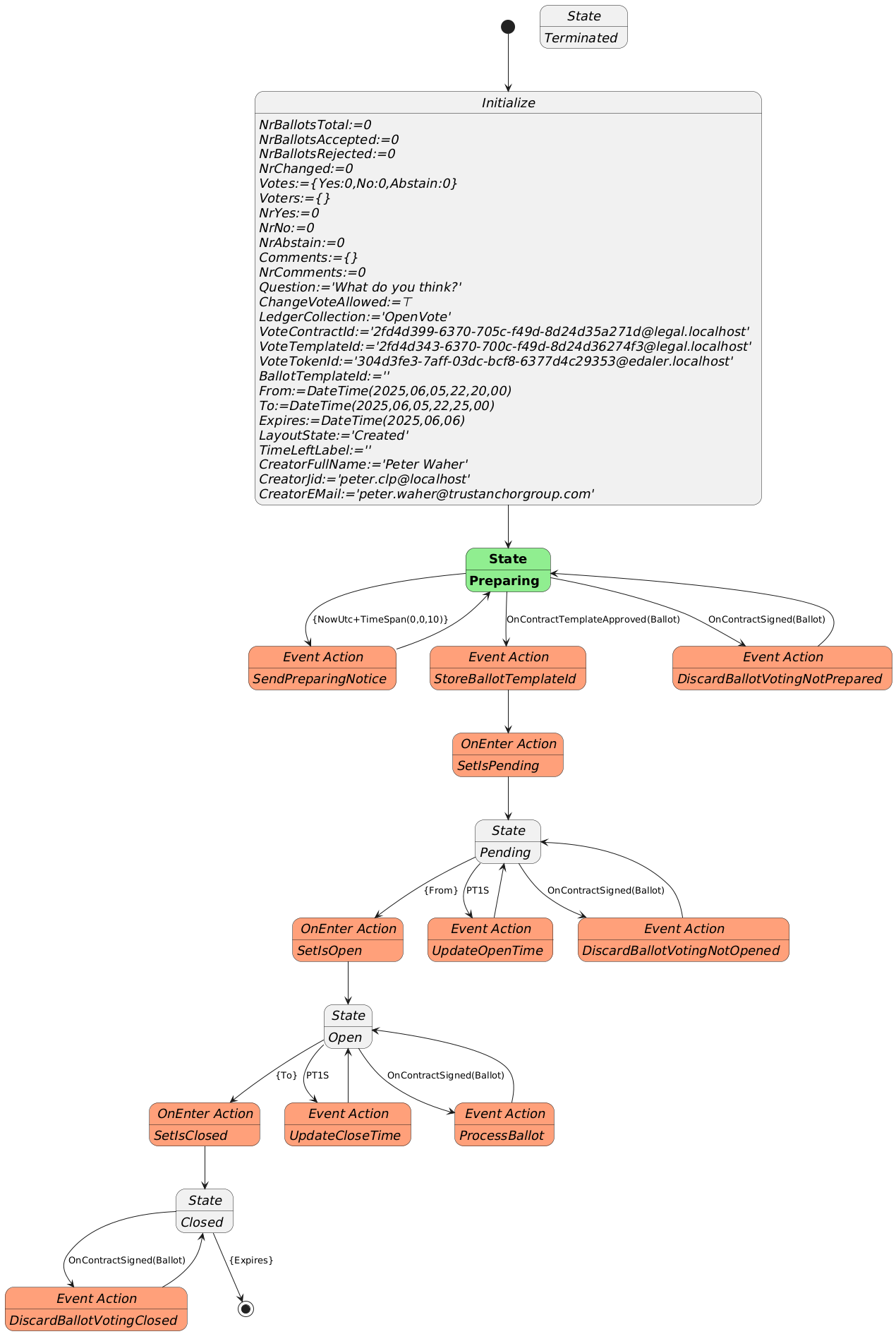
The voting contract allows the creator to pose a question, define if participants are allowed to change their votes, and between what times the vote will be active. Times are defined in UTC. Participants need personal digital IDs with at least a validated country code, personal number and full name. Only one ballot per (Country, Country Code) will be counted. Apart from a Creator, the contract also requires a Certifier, which can be the same as the creator. The Certifier are given rights to cancel (invalidate) an election.
The states a voting procedure passes through are:
During the Preparing state, the state machine checks if it is paired with a Ballot template that points to it. The Ballot template needs to define a contract reference parameter to the vote, and it needs to enforce the template of the vote to match the template used to create the vote. If one such Ballot template is found, it progresses automatically to the next state. If one is not found, it awaits until such a template is approved on the Neuron®.
The Pending state, is a state where the vote is prepared, but has not commenced yet. During this state, the present view can be displayed, and links to the vote can be distributed. Any ballots cast during this state will be automatically rejected. Once the time to open the vote has been reached, the state-machine progresses to the next state.
The Open state is where participants can vote by signing Ballot contracts. If they are signed using the appropriate template, and pointing to the vote, the state-machine will process the ballots. If any errors are encountered, the corresponding ballot is rejected, and error logged. When the finishing time has been reached, the state-machine progresses to the next state.
The last state is the Closed state. Here, the voting machine is kept alive, to present results, but no ballots are processed, so the tally cannot be changed. Once the expiry time is reached, the state-machine is ended.
During state changes, the creator is notified by XMPP (Instant Chat Message) and e-Mail about the state of the vote. The messages contain links to the vote. These can be distributed to participants of the vote. The vote can also be shown in a browser. If published, voters and other participants can follow the results in real-time.
Ballot Contract
The second contract template is the OpenBallotYesNoAbstain.xml contract. It defines the ballot contract that can be used to participate in any votes generated by the corresponding voting template. The two templates must be paired. So, once the voting template has been proposed and apporved, its ID must be set into the Ballot template contract, before it can be proposed. Once this has been done, it can be proposed and approved accordingly. Then, the pair can be used with as many votes as desired.
The Ballot template defines the Boolean parameters necessary to be able to select Yes, No or Abstain. The Machine-readable part (defined by the https://paiwise.tagroot.io/Schema/OpenVote.xsd
namespace) instructs the vote state-machine how the ballot is to be interpreted. The Ballot template has a contract reference parameter of a given name, and it must require a contract reference having a template ID restriction equal to the ID of the voting template. Once the voting state-machine is running, it will provide links to the corresponding Ballot contract template, with these references pre-set, so the user does not need to worry about providing the values for thse references. The user will only scan a QR code to vote.
Approved templates for experimentation
If you want to experiment with the open voting solution described, you can use the following approved contract templates. You can either copy the contract IDs and use in your application, or if you use an App, scan the corresponding QR code to access it.
To create a vote, i.e. define a question partiticpants will vote on, use the following template by scanning the code or entering its ID in the appropriate interface:
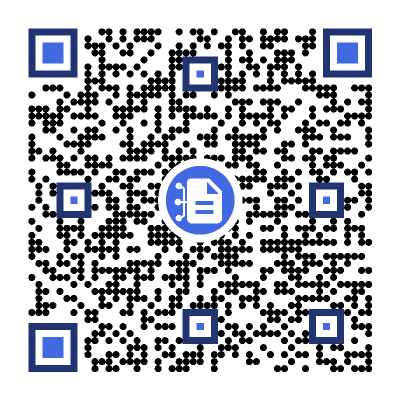
The matching Ballot template (which you will not need to scan here directly, as it will be presented pre-filled for you, see below), is:
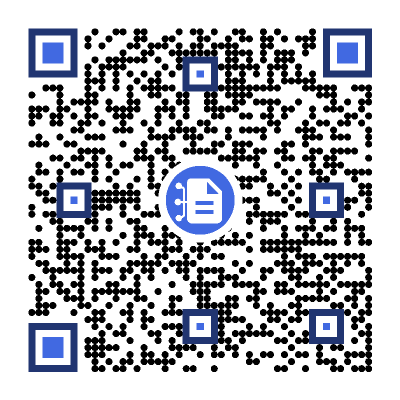
After scanning the code, a new vote contract will be displayed. Fill in the details (question, if votes can be changed and the time interval the vote will be open; remember the times need to be provided in Universal Time Coordinates, or UTC). Sign the contract, both as Creator and Certifier (unless you want another person to sign as Certifier). Once the vote has been created and been properly signed, a Neuro-Feature token will be created. It will send you an e-mail with a link to the voting results page. The vote only passes slightly the preparation state, as an approved ballot template already exists. The vote page will look something as follows. Note the QR code to the right. It will point to the ballot contract template to use to cast a ballot, and also contain the reference to the current vote pre-filled. All you need to do after scanning the QR-code, is to select how you want to vote.
Note: The vote token is defined to be unique. This means, you can only create one token (one vote) with the same set of parameters. This means, you need to change any of the parameters from previous versions, including the question or time parameters, before you can create additional votes.
Vote Count & real-time presentation
The token that is generated by the vote contract has a present report that displays the current state of the vote. The creator can access the present report from the client used to create the vote contract, and by extension the token. The creator will also get an Instant Chat Message and an e-Mail, containing the present report and current vote count (which would be zero and pending, before the count starts). There will also be a web URL which can be distributed, that will show the vote to any online viewer. The URL will have a format similar to https://sa.id.tagroot.io/NF/a86d6033-b694-7b5e-c8d3-42d5200ec85c@edaler.sa.id.tagroot.io
. The ID in the URL corresponds to the ID of the Vote. You can match this ID with the ID displayed for the vote (see below). The present report can be projected and displayed in kiosk mode as well. It will be updated in real-time as votes are counted. The initial view may be something as follows:
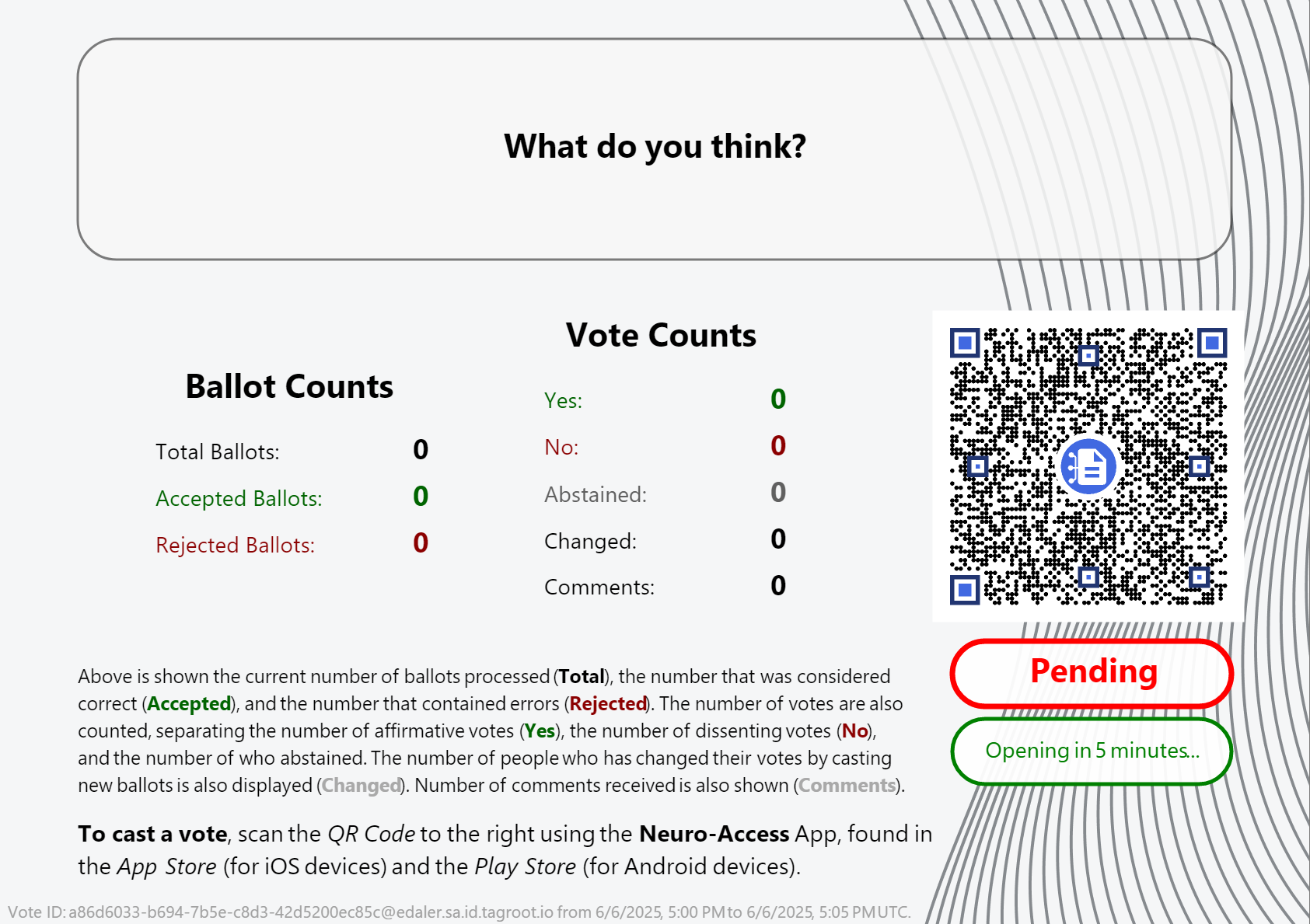
Note The QR code in the report can be scanned by participants, and will point to the ballot template, with the vote reference pre-filled in. All the participant needs to do, is vote, and sign the ballot and the vote will be counted. Once the vote closes, the report will be updated accordingly also.
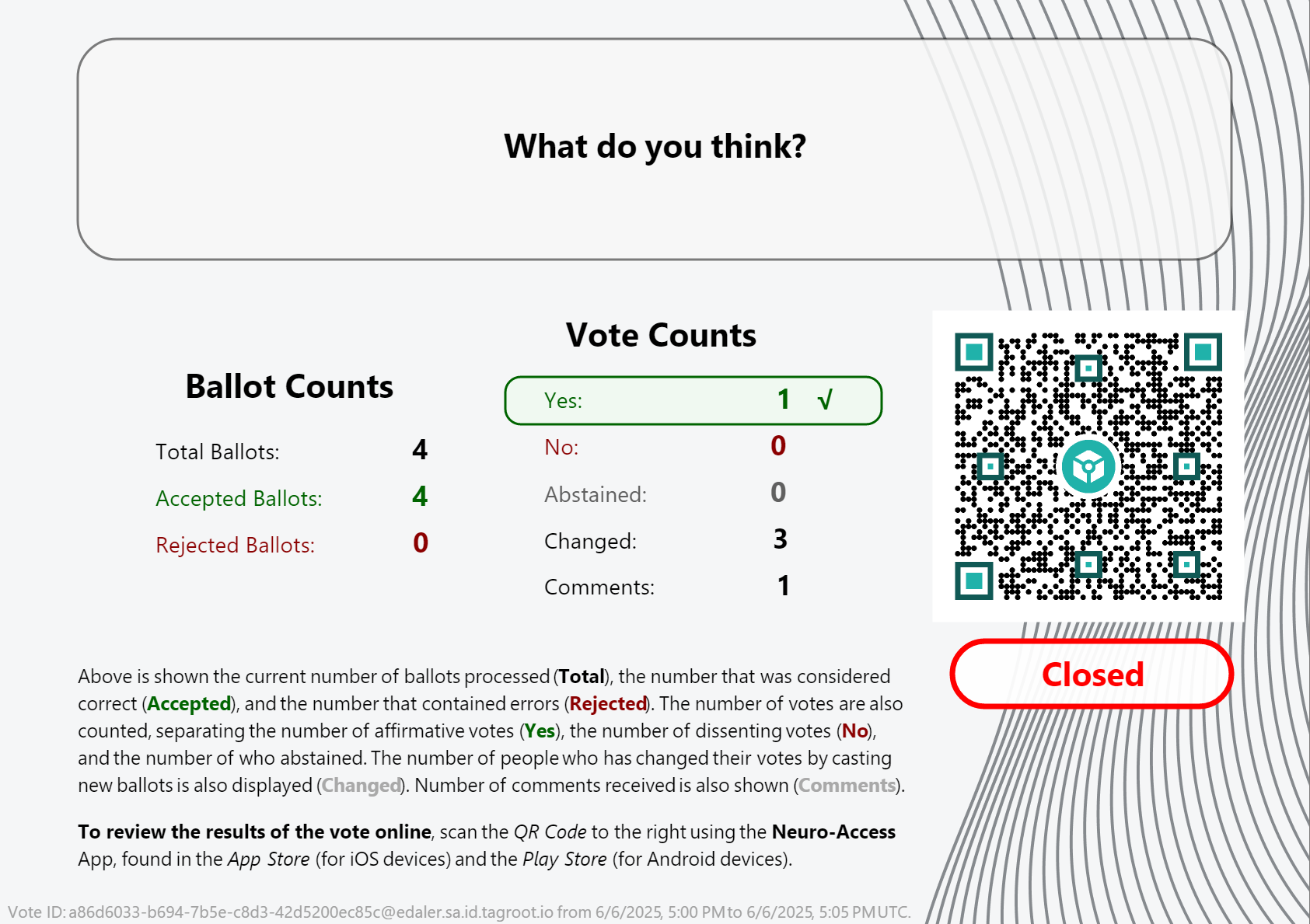
Note: Once the vote closes, the QR code changes. It is no longer pointing to the ballot template, but instead to the token that performed the count. Scanning it will give access to the token, and the reports it publishes.
Presentation Layout
The layout presenting the vote count is generated by the voting token itself, and is part of the vote contract template, and can therefore be reviewed as well. It is an XML Layout format, availble in the OpenVoteResultLayout.xml
file. You can edit such XML files and preview the layout using LegalLab as follows:
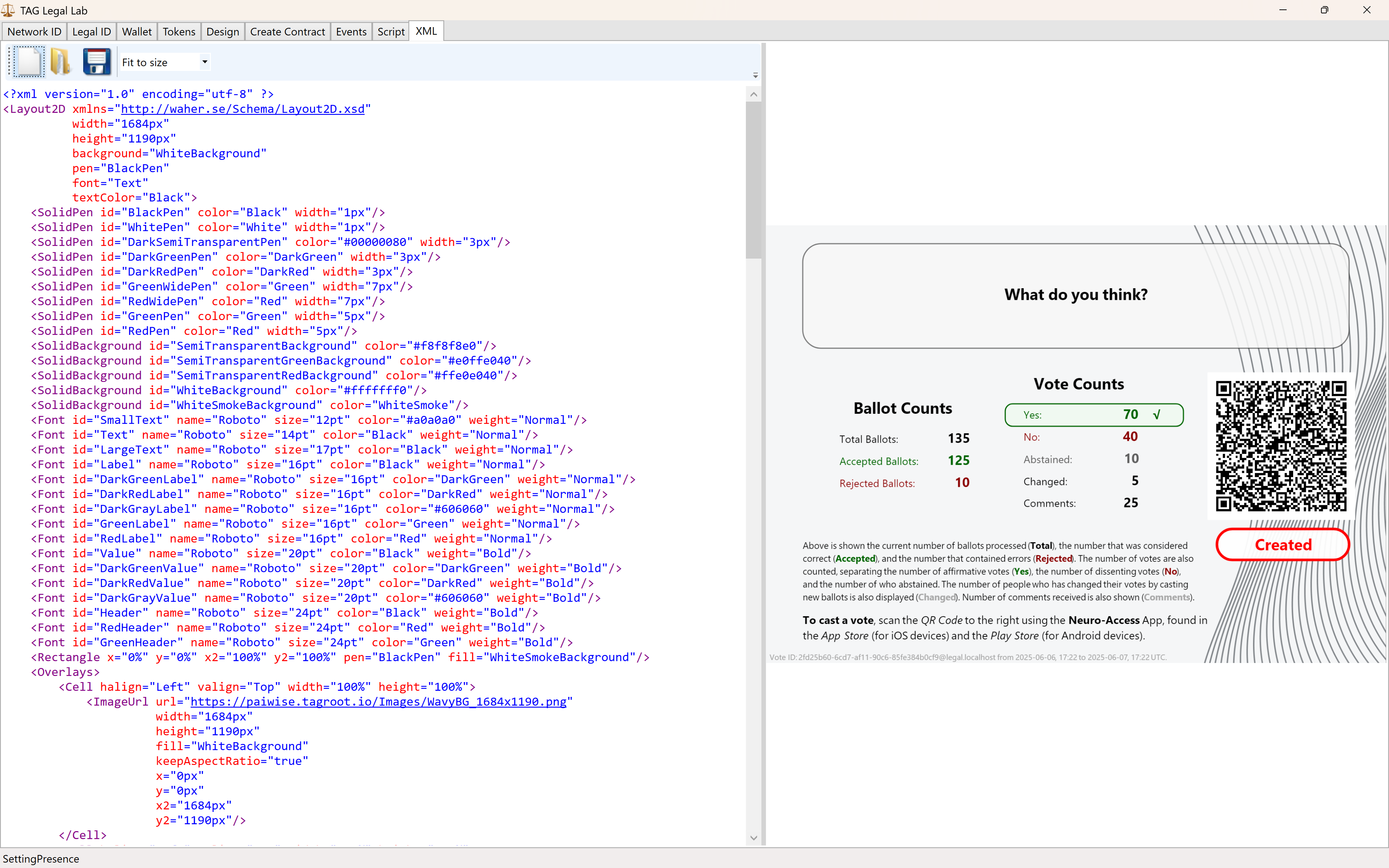
The variables and script functions used in the layout need to be initialized. This can be done in the Script tab. You can use the initialization script published in the repository: OpenVoteResultLayoutInit.script
to generate the view above.
Neuro-Ledger entries recorded
Apart from all entries recorded regarding Digital Identities, Smart Contracts, Tokens and State-Machines, the Open Vote example records several entries by itself. These include:
Entry | Description |
---|---|
BallotCounted |
A ballot has been counted. |
BallotRejected |
A ballot has been rejected. |
BallotRevoked |
When an older ballot has been revoked because the participant submits a new ballot. |
CommentReceived |
A comment by a participant has been received. |
Note: The vote contract defining the vote, also defines the Neuro-Ledger Collection these entries are recorded in.
The other common Neuro-Ledger collections annotating entries are:
Neuro-Ledger Collections | |
---|---|
LegalIdentities |
Contains entries related to digital identities, including Neuro-Access digital identities. |
Contracts |
Contains entries related to smart contracts, including the voting contract and the ballot contracts used during voting. |
NeauroFeatureTokens |
Contains information regarding Neuro-Feature tokens, including the token definition of the open voting logic. |
StateMachines |
State-Machine information is recorded in this collection. This includes the open voting process performing the ballot counting and presentation. |
StateMachineCurrentStates |
Contains current states of different State-Machines. |
StateMachineSamples |
Contains variable changes as they are persisted by State-Machines. These samples can be used to track the counting process. |
#tutorial, #example, #legallab, #contracts, #neuro-feature, #neuro-ledger, #state-machine
Resending Verification Codes during Onboarding
The TAG ID Onboarding API has been updated to allow for resending verification codes, without generating new codes. There are two parts of this API:
For clients that access an onboarding Neuron®, the
/ID/SendVerificationMessage.ws
resource has been updated to allow for resending codes, by providing anResend
property in the request. If set totrue
, the resource will resend any existing code to the registered components, otherwise an error will be returned.For clients using the Agent API, a new resource is available, permitting the resending of verification codes:
/Agent/Account/ResendVerificationCodes
.
Onboarding API
The /ID/SendVerificationMessage.ws
now accepts payload having the following format:
{
Nr:Optional(Str(PNr like "\\+[1-9]\\d+")),
EMail:Optional(Str(PEMail like "[\\w\\d](\\w|\\d|[_\\.-][\\w\\d])*@(\\w|\\d|[\\.-][\\w\\d]+)+")),
AppName:Optional(Str(PAppName)),
Language:Optional(Str(PLanguage)),
Resend:Optional(Bool(PResend))
}
If the Resend
property is available, and is true
, the method will resend any existing code to the existing number or e-mail address provided. It is not possible to send an existing code to a new number or e-mail address.
Agent API
The Agent API (from build 2025-06-02
) now has a new resource: /Agent/Account/ResendVerificationCodes
. This resource allows for the resending of verification codes for the account currently being created. In order to access it, the JSON Web Token (JWT) provided in the response when creating the resource must be provided. The caller also needs to provide the phone number and/or e-mail address to which codes should be resent.
Security Notice: It is not possible to resend codes for accounts, numbers or e-mail addresses that have been verified. You can only resend codes for accounts still pending verification. This includes partially verified accounts. If the phone number has been verified, but the e-mail address has not, or vice versa, you can resend the code for the unverified part, but not for the verified part. Attempting to resend codes that have been verified, will be flagged, and repetetive calls to resend codes for verified accounts, numbers or addresses may result in the temporary and then permanent blocking of the endpoint making the call.
#new, #api, #onboarding, #id
IP Location Information during Onboarding
The Onboarding API has been updated to provide some additional IP Location information during onboarding, to help clients prefill fields in ID applications.
When calling the https://id.tagroot.io/ID/CountryCode.ws
web service (using POST, and Accept
header set to application/json
), the following information will now be available in the response:
{
"RemoteEndPoint": string,
"CountryCode": string,
"PhoneCode": string,
"Country": string,
"Region": string,
"City": string,
"Latitude": double,
"Longitude": double
}
The IP Location information is provided by IP2Location.
Note: The information provided in the response may be incorrect, so users will need to verify the information provided, before including it in any ID applications.
#new, #api, #onboarding, #id
Modbus-XMPP Bridge
The IoTBridgeModbus repository provides an IoT bridge between devices connected to Modbus, for use in closed intra-networks or enterprise networks, and the harmonized XMPP-based Neuro-Foundation network, for open and secure cross-domain interoperation on the Internet.
To run the bridge, you need access to both a Modbus gateway, and an XMPP broker, that supports the Neuro-Foundation extensions. You can use the TAG Neuron for XMPP.
Running and configuring the bridge
The code is written using .NET Standard, and compiled to a .NET Core console application that can be run on most operating systems. Basic configuration is performed using the console interface during the first execution, and persisted. You can also provide the corresponding configuration using environment variables, making it possible to run the bridge as a container. If an environmental variable is missing, the user will be prompted to input the value on the console.
Environmental Variable | Type | Description |
---|---|---|
XMPP_HOST |
String | XMPP Host name |
XMPP_PORT |
Integer | Port number to use when connecting to XMPP (default is 5222 ) |
XMPP_USERNAME |
String | User name to use when connecting to XMPP. |
XMPP_PASSWORD |
String | Password (or hashed password) to use when connecting to XMPP. Empty string means a random password will be generated. |
XMPP_PASSWORDHASHMETHOD |
String | Algorithm or method used for password. Empty string means the password is provided in the clear. |
XMPP_APIKEY |
String | API Key. If provided together with secret, allows the application to create a new account. |
XMPP_APISECRET |
String | API Secret. If provided together with key, allows the application to create a new account. |
MODBUS_HOST |
String | Modbus Gateway Host name |
MODBUS_TLS |
String | If TLS encryption is to be used when connecting to the Modbus gateway. |
MODBUS_PORT |
String | Port number to use when connecting to the Modbus gateway (default is 502 ). |
REGISTRY_COUNTRY |
String | Country where the bridge is installed. |
REGISTRY_REGION |
String | Region where the bridge is installed. |
REGISTRY_CITY |
String | City where the bridge is installed. |
REGISTRY_AREA |
String | Area where the bridge is installed. |
REGISTRY_SRTEET |
String | Street where the bridge is installed. |
REGISTRY_STREETNR |
String | Street number where the bridge is installed. |
REGISTRY_BUILDING |
String | Building where the bridge is installed. |
REGISTRY_APARTMENT |
String | Apartment where the bridge is installed. |
REGISTRY_ROOM |
String | Room where the bridge is installed. |
REGISTRY_NAME |
String | Name associated with bridge. |
REGISTRY_LOCATION |
Boolean | If location has been completed. (This means, any location-specific environment variables not provided, will be interpreted as intensionally left blank, and user will not be prompted to input values for them. |
Claiming ownership of bridge
Once the bridge has been configured, it will generate an iotdisco
URI, and save it to its programd data folder. It will also create a file with extension .url
, containing a shortcut with the iotdisco
URI inside. A .png
file with a QR code will also be generated. All three files contain information about the bridge, and allows the owner to claim ownership of it. This can be done by using the Neuro-Access App. This app is also downloadable for Android and iOS. You scan the QR code (or enter it manually), and claim the device. Once the device is claimed by you, you will receive notifications when someone wants to access the deice. They will only be able to access it with the owner’s permission. For more information, see:
Configuring the bridge
The bridge can be configured in detail by a client that implements the concentrator interface. Concentrators consist of data sources, each containing tree structures of nodes. Nodes may be partitioned into partitions, which permits the nesting of subsystems seamlessly into container systems. Each node can be of different types, and have different properties and underlying functionality. They can each implement then sensor interface and actuator interface.
You can use the Simple IoT Client to configure concentrators and their nodes in detail. An initial setup is done using the initial configuration of the bridge. The client is also available in the IoTGateway repository, in the Clients folder.
Node Types
The bridge includes several different node types that can be used to configure its operation:
The
Modbus Gateway
maintains a connection to a Modbus Gateway using TCP/IP, and converts requests into binary Modbus TCP/IP communication.The
XMPP Broker
maintains a connection to an XMPP Broker. It allows the bridge to connect to other entities on the federated network and communicate with them. It supports communication with remote standalone sensors and actuators, as well as remote concentrators embedding devices into data sources and nodes. Such concentrators can be bridges to other protocols and networks.Note: The bridge has a client-to-server connection by default, setup during initial configuration. Through this connection, the bridge acts as a concentrator. Through the use of
XMPP Broker
nodes you can setup additional XMPP connections to other brokers. In these cases the bridge will only act as a client, to connect to remove devices for the purposes of interacting with them.IP Host
nodes allow you to monitor network hosts accessible from the bridge.Script
nodes allow you to create nodes with custom script logic. They can be used to interface bespoke devices in the network accessible from the bridge, for example.Virtual
nodes are placeholders where external logic (or script logic) can aggregate information in a way that makes them accessible by others in the federated network.
Posts by user
No more posts authored by the user could be found. You can go back to the main view by selecting Home in the menu above.